In Python, variables play a crucial role in storing and manipulating data. They act as containers that hold values. In this article, we’lll be discussing about the fundamentals of variables in Python.
What are Variables?
Variables are names assigned to data values in Python. They act as placeholders, allowing us to refer to the data by its assigned name. Think of variables as labeled boxes where you can store different types of information.
Variable Naming Conventions and Rules
Python has specific rules and conventions for naming variables. Following these guidelines ensures code clarity and maintainability. Here are some key points to remember:
Variable names can contain letters, numbers, and underscores (_), but they must start with a letter or an underscore.
Variable names are case-sensitive, meaning “myVar” and “myvar” are treated as different variables.
Avoid using reserved words (keywords) as variable names, such as if, while, or def.
Choose meaningful and descriptive names to enhance code readability.
Assigning Values to Variables
To assign a value to a variable, we use the assignment operator (=). Here’s an example:
myVariable = 42
In the above example, we assigned the value 42 to the variable “myVariable”.
Variable Reassignment and Updating Values
In Python, variables can be reassigned with new values. This means you can change the value of a variable after it has been assigned.
Here’s an example:
x = 10 x = x + 5
In the above example, we initially set x to 10, and then we reassign it by adding 5 to its existing value. The final value of x becomes 15.
Variable Scope
Variables in Python have scope, which defines their visibility and accessibility within different parts of the code. The scope determines where a variable can be accessed and modified.
The two main types of variable scope in Python are:
- Global scope: Variables defined outside of any function or class have global scope and can be accessed from anywhere in the code.
- Local scope: Variables defined within a function or block have local scope and can only be accessed within that specific scope.
Understanding variable scope is crucial for writing maintainable and bug-free code.
Frequently Asked Questions
Below, you can find the answers to some relevant questions on Python variables.
How does variable scoping work in Python when dealing with nested functions or classes?
Variable scoping in Python follows the LEGB rule, which stands for Local, Enclosing, Global, and Built-in scopes. When dealing with nested functions or classes, Python searches for variables in the following order: local scope (inside the function), enclosing scope (outside but enclosing the function), global scope (outside any function), and finally built-in scope (predefined Python functions and objects).
Are there any performance considerations when frequently reassigning variables in Python?
Frequent variable reassignment can have performance implications in Python, especially in performance-critical applications or when dealing with large datasets. Each reassignment involves memory allocation and deallocation, which can lead to increased overhead. Additionally, excessive reassignment may hinder code readability and maintainability. It’s essential to consider alternative approaches, such as using immutable objects or optimizing algorithms, to mitigate potential performance issues.
What are the best practices for managing variable scope to avoid potential bugs and unintended side effects?
To manage variable scope effectively and minimize potential bugs and unintended side effects, adhere to the following best practices:
- Limit the scope of variables to the smallest possible scope required for their usage.
- Avoid using global variables unless absolutely necessary, as they can lead to code coupling and make debugging challenging.
- Use descriptive variable names to enhance code readability and maintainability.
- Understand and leverage Python’s scoping rules, such as the LEGB rule, to ensure proper variable resolution.
- Consider using functions and classes to encapsulate variables and logic, promoting modular and reusable code.
- Use tools like linters and static code analyzers to identify potential scope-related issues early in the development process.
- Document variable scope and usage patterns to aid comprehension for yourself and other developers working on the codebase.
Conclusion
Variables are essential building blocks in Python programming. They allow us to store, manipulate, and retrieve data. By understanding variable naming conventions, assignment, reassignment, and scope, you’ll be equipped to write more robust and readable Python code.
Learn how to work with Python and SQL Server – Enroll to our course!
Enroll to our online course “Working with Python on Windows and SQL Server Databases” with an exclusive discount, and get started with Python data access programming for SQL Server databases, fast and easy!
By the time you complete the course:
- You will know how to install Python on Windows and set your development environment with Visual Studio Code and the proper extensions
- You will know how to connect your Python programs to SQL Server instances and databases
- You will know how to run different types of T-SQL queries against your SQL Server databases, from within your Python Programs
- Execute and process SELECT, INSERT, UPDATE and DELETE T-SQL statements
- Call Dynamic Management Views (DMVs)
- Call aggregation functions
- Call SQL Server global system variables
- You will know how to execute SQL Server functions and stored procedures, from within your Python Programs
- You will know how to use parameters and exception handling for all the above database operations within your Python code
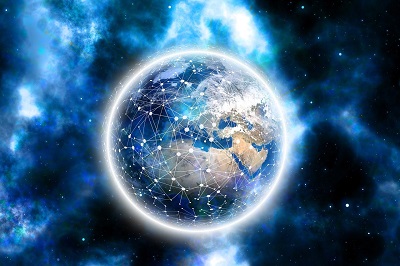
Recommended Online Courses:
- AI Essentials: A Beginner’s Guide to Artificial Intelligence
- Human-AI Synergy: Teams and Collaborative Intelligence
- SQL Server 2022: What’s New – New and Enhanced Features
- Working with Python on Windows and SQL Server Databases
- Introduction to Azure Database for MySQL
- Boost SQL Server Database Performance with In-Memory OLTP
- Introduction to Azure SQL Database for Beginners
- Essential SQL Server Administration Tips
- SQL Server Fundamentals – SQL Database for Beginners
- Essential SQL Server Development Tips for SQL Developers
- Introduction to Computer Programming for Beginners
- .NET Programming for Beginners – Windows Forms with C#
- SQL Server 2019: What’s New – New and Enhanced Features
- Entity Framework: Getting Started – Complete Beginners Guide
- Data Management for Beginners – Main Principles
- A Guide on How to Start and Monetize a Successful Blog
Read Also:
- Main Data Structures in Python
- SyntaxError: invalid syntax when using IF in Python – How to Resolve it
- IndexError: list index out of range – How to Resolve it in Python
- Input and Output in Python
- How to Write a “Hello World” App in Visual C++
- How to Write a “Hello World” App in C#
- How to Get Started with SQL Server – First Steps
- Benefits of Primary Keys in Database Tables
- How to Rebuild All Indexes Online for a SQL Server Database
- What’s the Best Allocation Unit Size when Formatting a USB Flash Drive?
Reference: {essentialDevTips.com} (https://www.essentialdevtips.com/)
© essentialDevTips.com
Rate this article:
Artemakis Artemiou is a Senior SQL Server Architect, Author, a 9 Times Microsoft Data Platform MVP (2009-2018). He has over 15 years of experience in the IT industry in various roles. Artemakis is the founder of SQLNetHub and {essentialDevTips.com}. Artemakis is the creator of the well-known software tools Snippets Generator and DBA Security Advisor. Also, he is the author of many eBooks on SQL Server. Artemakis currently serves as the President of the Cyprus .NET User Group (CDNUG) and the International .NET Association Country Leader for Cyprus (INETA). Moreover, Artemakis teaches on Udemy, you can check his courses here.