Hi friends! In this article, we will be discussing about, how you can use Dynamic Memory Allocation in Java, and see a relevant code example.
What is Dynamic Memory Allocation in Java?
In Java, Dynamic Memory Allocation, is the ability for a program, to obtain more memory space during it’s execution when needed (i.e. create instantiate a new class object), as well as, to release this space when is no longer needed.
Is there a Limit for Dynamic Memory Allocation in Java?
Programmatically, there is no limit for Dynamic Memory Allocation in Java. The only limit has to do with the actual physical memory on the machine that the java code executes. By saying “physical memory” we mean both RAM and virtual memory.
When does Dynamic Memory Allocation Occur in Java?
Dynamic Memory Allocation occurs, when the “new” keyword is used in Java code.
For example, if you have a class named “Node”, whenever you instantiate a new object for the class using the below – or similar – code, then the required amount of memory is dynamically allocated in order to be able to create the object:
Node newNode1=new Node();
Full Code Example of Dynamic Memory Allocation in Java
Below, you can check an example prepared for this article, that demonstrates Dynamic Memory Allocation in Java.
As you can see in the below example, under the main class, I’m declaring a new class named “Node” that has two attributes, that is “nodeName” and “nodeData”.
Also, I’m specifying the constructor for my class, that takes two input parameter, used for populating with data the class’s attributes.
Then, in the “main” method of my Java program, I instantiate the “Node” class, by creating 3 new objects of the class. These three new objects are named:
- newNode1
- newNode2
- newNode3
Last, each time I create a new object of the “Node” class, I print on screen its two attribute data, just to confirm that the object has been properly constructed.
Here’s the code:
class TestDynamicMemAllocation { static class Node { String nodeName; String nodeData; public Node(String prmNodeName, String prmNodeData) { this.nodeName = prmNodeName; this.nodeData = prmNodeData; } } public static void main(String args[]) { Node newNode1 = new Node("node1", "nodeData1"); System.out.println(newNode1.nodeName + " - " + newNode1.nodeData); Node newNode2 = new Node("node2", "nodeData2"); System.out.println(newNode2.nodeName + " - " + newNode2.nodeData); Node newNode3 = new Node("node3", "nodeData3"); System.out.println(newNode3.nodeName + " - " + newNode3.nodeData); } }
Handling the error: No enclosing instance of type … is accessible
In the above example, I have used the “static” keyword for the “Node” class, since I chose to include this class in the same .java file with the main class of the program. If I didn’t use the “static” keyword, I would get the below error message:
No enclosing instance of type TestDynamicMemAllocation is accessible. Must qualify the allocation with an enclosing instance of type TestDynamicMemAllocation (e.g. x.new A() where x is an instance of TestDynamicMemAllocation).
As mentioned above, one way to overcome this issue, is to use the “static” keyword. Alternatively, you can declare the “Node” class in a separate .java file.
Running the Code Example
Below, you can check the output of my code, when executed in Visual Studio Code:
Learn more about Programming – Enroll to our Course!
Enroll to our course on Udemy, titled “Introduction to Computer Programming for Beginners” and get the help you need for getting started with C++, C, Python, SQL, Java, C# and the main phases of the Software Development Lifecycle.
By the time you complete this course:
- You will know what is the required skill set in order to become a great Computer Programmer.
- You will know more about the Programmer’s mindset.
- You will know the main programming principles and fundamentals.
- You will know the main phases of the Software Development Life Cycle (SDLC).
- You will be able to start working with the following programming languages and write simple programs: C#, SQL, Java, C, C++ and Python.
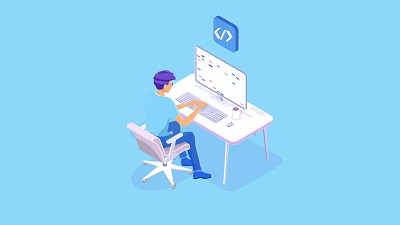
Recommended Online Courses:
- SQL Server 2022: What’s New – New and Enhanced Features
- Introduction to Azure Database for MySQL
- Working with Python on Windows and SQL Server Databases
- Boost SQL Server Database Performance with In-Memory OLTP
- Introduction to Azure SQL Database for Beginners
- Essential SQL Server Administration Tips
- SQL Server Fundamentals – SQL Database for Beginners
- Essential SQL Server Development Tips for SQL Developers
- Introduction to Computer Programming for Beginners
- .NET Programming for Beginners – Windows Forms with C#
- SQL Server 2019: What’s New – New and Enhanced Features
- Entity Framework: Getting Started – Complete Beginners Guide
- A Guide on How to Start and Monetize a Successful Blog
- Data Management for Beginners – Main Principles
Read Also:
- Main Data Structures in Python
- SyntaxError: invalid syntax when using IF in Python – How to Resolve it
- IndexError: list index out of range – How to Resolve it in Python
- Input and Output in Python
- How to Write a “Hello World” App in Visual C++
- How to Write a “Hello World” App in C#
- How to Get Started with SQL Server – First Steps
- Benefits of Primary Keys in Database Tables
- How to Rebuild All Indexes Online for a SQL Server Database
- What’s the Best Allocation Unit Size when Formatting a USB Flash Drive?
Reference: {essentialDevTips.com} (https://www.essentialdevtips.com/)
© essentialDevTips.com
Rate this article:
Artemakis Artemiou is a Senior SQL Server Architect, Author, a 9 Times Microsoft Data Platform MVP (2009-2018). He has over 15 years of experience in the IT industry in various roles. Artemakis is the founder of SQLNetHub and {essentialDevTips.com}. Artemakis is the creator of the well-known software tools Snippets Generator and DBA Security Advisor. Also, he is the author of many eBooks on SQL Server. Artemakis currently serves as the President of the Cyprus .NET User Group (CDNUG) and the International .NET Association Country Leader for Cyprus (INETA). Moreover, Artemakis teaches on Udemy, you can check his courses here.